题目
本题有两个不同难度的版本:139. Word Break 和 140. Word Break II。
139. Word Break
- Difficulty: Medium
- Total Accepted: 263K
- Total Submissions: 798K
Given a non-empty string s and a dictionary wordDict containing a list of non-empty words, determine if s can be segmented into a space-separated sequence of one or more dictionary words.
Note:
The same word in the dictionary may be reused multiple times in the segmentation.
You may assume the dictionary does not contain duplicate words.
Example 1:
Input: s = "leetcode", wordDict = ["leet", "code"]
Output: true
Explanation: Return true because "leetcode" can be segmented as "leet code".
Example 2:
Input: s = "applepenapple", wordDict = ["apple", "pen"]
Output: true
Explanation: Return true because "applepenapple" can be segmented as "apple pen apple".
Note that you are allowed to reuse a dictionary word.
Example 3:
Input: s = "catsandog", wordDict = ["cats", "dog", "sand", "and", "cat"]
Output: false
140. Word Break II
- Difficulty: Hard
- Total Accepted: 133K
- Total Submissions: 520K
Given a non-empty string s and a dictionary wordDict containing a list of non-empty words, add spaces in s to construct a sentence where each word is a valid dictionary word. Return all such possible sentences.
Note:
The same word in the dictionary may be reused multiple times in the segmentation.
You may assume the dictionary does not contain duplicate words.
Example 1:
Input:
s = "catsanddog"
wordDict = ["cat", "cats", "and", "sand", "dog"]
Output:
[
"cats and dog",
"cat sand dog"
]
Example 2:
Input:
s = "pineapplepenapple"
wordDict = ["apple", "pen", "applepen", "pine", "pineapple"]
Output:
[
"pine apple pen apple",
"pineapple pen apple",
"pine applepen apple"
]
Explanation: Note that you are allowed to reuse a dictionary word.
Example 3:
Input:
s = "catsandog"
wordDict = ["cats", "dog", "sand", "and", "cat"]
Output:
[]
解题报告
139. Word Break
AC 截图
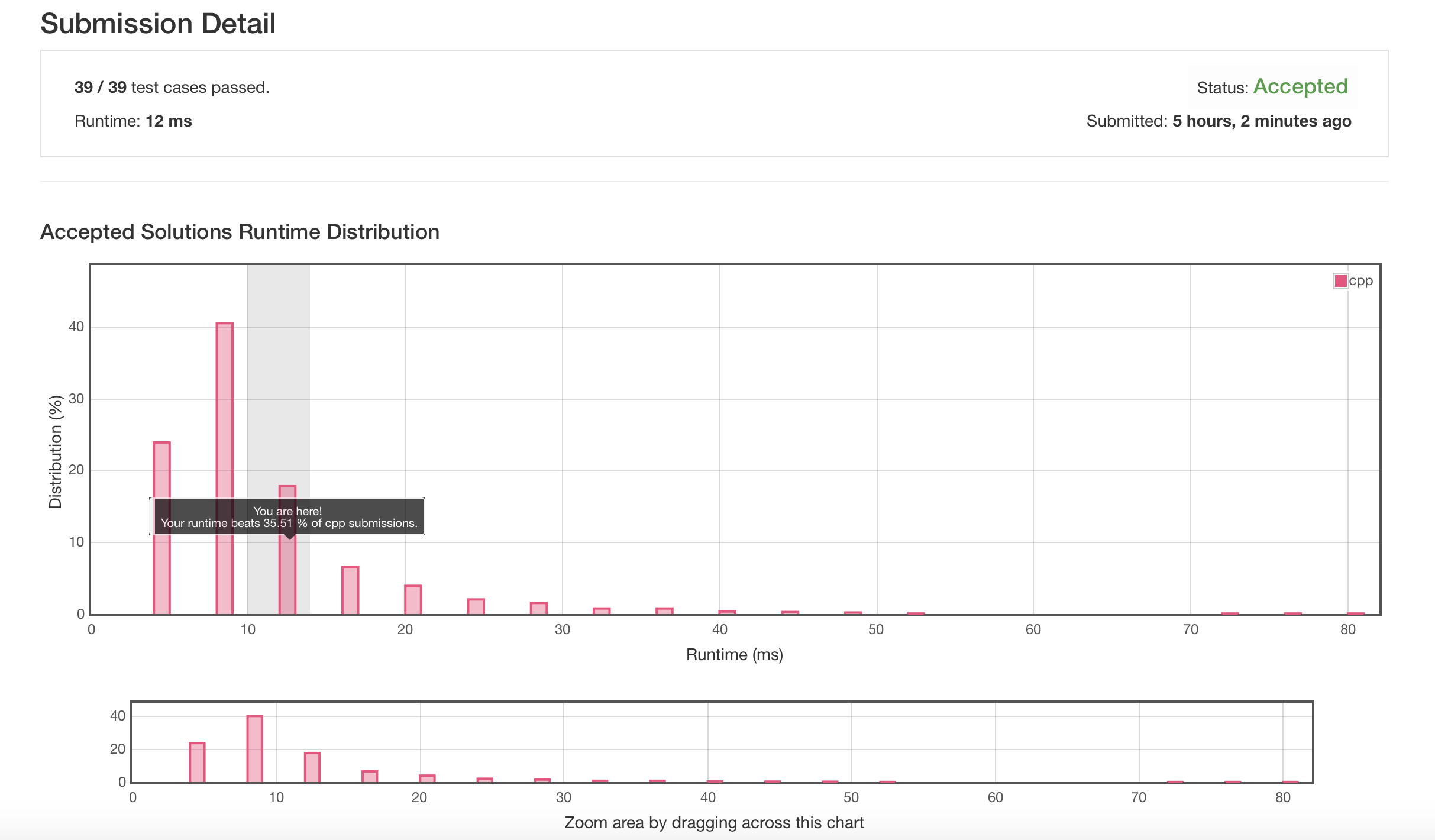
题目大意
给出一个非空字符串,判断其是否能够由字典中的单词组成。
解题思路
设置一个布尔数组,对于其中下标为 i 的项,表示从起始字符到第 i 个字符为一个合法子串。其中,下标为 0 初始为 true
。通过如下方式更新这个布尔数组:
对于下标为 i 的项,向前遍历布尔数组,如果第 j 项为 true,则判断从 j 到 i 的子字符串是否在字典内。若在字典内,则当前项置 true,退出内循环,并且继续向第 i + 1 项遍历更新。
在遍历完后,我们只需判断最后一项是否为 true
即可。
题解
1 | class Solution { |
140. Word Break II
AC 截图
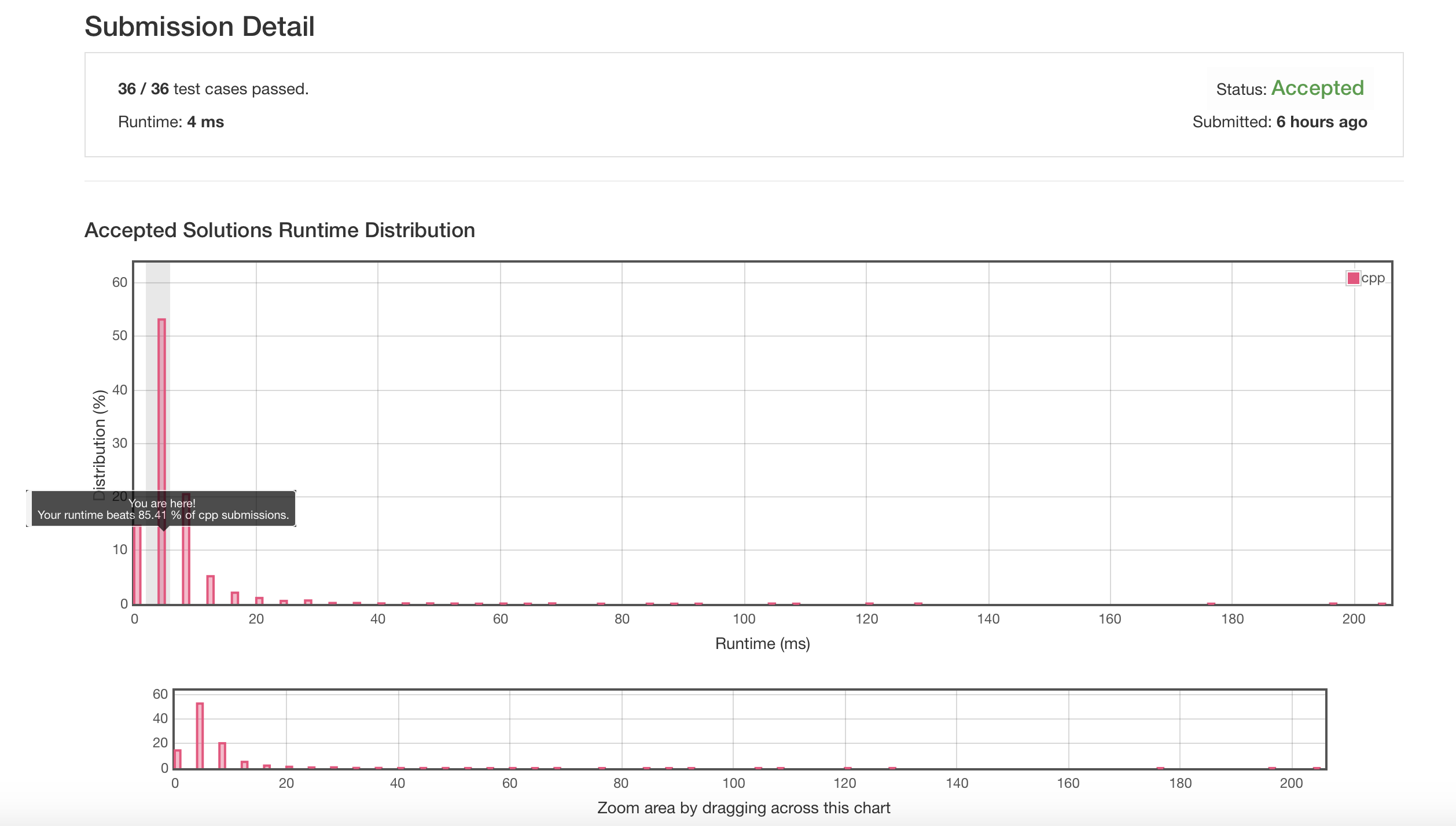
题目大意
和上题基本一致,但是本题额外要求我们输出所有可能的分割情况。
解题思路
把布尔数组换成一个二维数组。然后在字典内找到当前项的时候,不退出内循环,但是将 j 添加到第 i 项的数组内。最后我们只需要通过这些数组重建字符串即可。
题解
1 | static const auto runfirst = []() { |